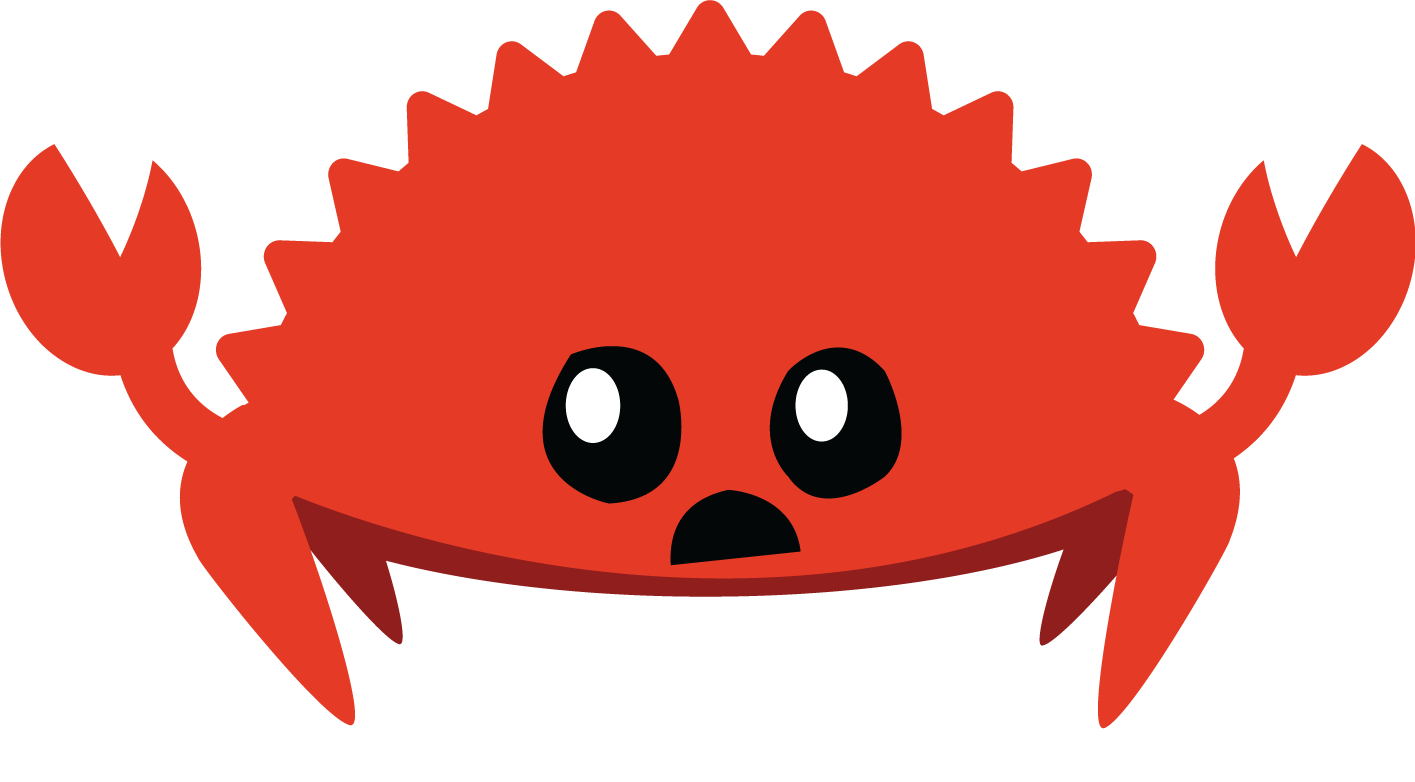
This past month, I have been enthralled by the Rust programming language
given its unique edge for writing memory-safe, modern programs. Over the years, several languages have
emerged as the most preferred by engineers to write resilient, backend software. The tides have shifted
from Java/C++ into Go and Rust, which combine decades of programming language theory to build tools
that are effective in our current age.
Rust’s numbers speak for themselves. As the number 1 most loved language in
the famous stack overflow survey for seven years in a row, it has also recently been
released as part of Linux kernel - a feat no language other than C has been able to
accomplish. What’s exciting about the language, to me, is that it provides something truly new
in the art of how software is built.
use std::thread;
use std::time::Duration;
use std::{collections::VecDeque, sync::Condvar, sync::Mutex};
fn main() {
let queue = Mutex::new(VecDeque::new());
thread::scope(|s| {
let t = s.spawn(|| loop {
let item = queue.lock().unwrap().pop_front();
if let Some(item) = item {
dbg!(item);
} else {
thread::park();
}
});
for i in 0.. {
queue.lock().unwrap().push_back(i);
t.thread().unpark();
thread::sleep(Duration::from_secs(1));
}
})
}